Справочник Жаркова по проектированию и программированию искусственного интеллекта. Том 6: Программирование на Visual Basic искусственного интеллекта. Продолжение 2
Шрифт:
currentBlock.Color)
End If
' look down
If row > 0 Then
nextRow = row – 1
selected = matrix(nextRow, column)
ExamineNeighbor(selected, nextRow, column, _
currentBlock.Color)
End If
' look left
If column > 0 Then
nextCol = column – 1
selected = matrix(row, nextCol)
ExamineNeighbor(selected, row, nextCol, _
currentBlock.Color)
End If
' look right
If column < matrix.GetLength(1) – 1 Then
nextCol = column + 1
selected = matrix(row, nextCol)
ExamineNeighbor(selected, row, nextCol, _
currentBlock.Color)
End If
End Sub
''' <summary>
''' If the neighbor is the same color, add it to the blocks
''' to examine.
''' </summary>
''' <param name="selected"></param>
''' <param name="row"></param>
''' <param name="column"></param>
''' <param name="color"></param>
''' <remarks></remarks>
Private Sub ExamineNeighbor(ByVal selected As Block, _
ByVal row As Integer, ByVal column As Integer, _
ByVal color As Color)
If Not selected Is Nothing Then
If selected.Color.Equals(color) Then
If Not selected.MarkedForDeletion Then
selected.MarkedForDeletion = True
blocksToExamine.Add(New Point(row, column))
End If
End If
End If
End Sub
End Class
По
Листинг 20.18. Новый файл.
''' <summary>
''' Represents one high score.
''' </summary>
''' <remarks></remarks>
Public Class HighScore
Implements IComparable
Public nameValue As String
Public scoreValue As Integer
Public Property Name As String
Get
Return nameValue
End Get
Set(ByVal Value As String)
nameValue = Value
End Set
End Property
Public Property Score As Integer
Get
Return scoreValue
End Get
Set(ByVal Value As Integer)
scoreValue = Value
End Set
End Property
Public Overrides Function ToString As String
Return Name & ":" & Score
End Function
Public Sub New(ByVal saved As String)
Name = saved.Split(":".ToCharArray)(0)
Score = CInt(saved.Split(":".ToCharArray)(1))
End Sub
Public Function CompareTo(ByVal obj As Object) As Integer Implements System.IComparable.CompareTo
Dim other As HighScore
other = CType(obj, HighScore)
Return Me.Score – other.Score
End Function
End Class
По
Листинг 20.19. Новый файл.
Imports Microsoft.Win32
''' <summary>
''' Reads and writes the top three high scores to the registry.
''' </summary>
''' <remarks></remarks>
Public Class HighScores
''' <summary>
''' Read scores from the registry.
''' </summary>
''' <returns></returns>
''' <remarks></remarks>
Public Shared Function GetHighScores As HighScore
Dim tops(2) As HighScore
Dim scoreKey As RegistryKey = Registry.CurrentUser. _
CreateSubKey("Software\VBSamples\Collapse\HighScores")
For index As Integer = 0 To 2
Dim key As String = "place" & index.ToString
Dim score As New HighScore(CStr(scoreKey.GetValue(key)))
tops(index) = score
Next
scoreKey.Close
Return tops
End Function
''' <summary>
''' Update and write the high scores.
''' </summary>
''' <param name="score"></param>
''' <remarks></remarks>
Public Shared Sub UpdateScores(ByVal score As Integer)
Dim tops(3) As HighScore
Dim scoreKey As RegistryKey = Registry.CurrentUser. _
CreateSubKey("Software\VBSamples\Collapse\HighScores")
tops(0) = New HighScore(scoreKey.GetValue("Place0").ToString)
tops(1) = New HighScore(scoreKey.GetValue("Place1").ToString)
tops(2) = New HighScore(scoreKey.GetValue("Place2").ToString)
If score > tops(2).Score Then
Dim name As String = InputBox("New high score of " & _
score & " for:")
tops(3) = New HighScore(" :0")
tops(3).Name = name
tops(3).Score = score
Array.Sort(tops)
Array.Reverse(tops)
scoreKey.SetValue("Place0", tops(0).ToString)
scoreKey.SetValue("Place1", tops(1).ToString)
scoreKey.SetValue("Place2", tops(2).ToString)
End If
scoreKey.Close
End Sub
''' <summary>
Измена. Жизнь заново
1. Измены
Любовные романы:
современные любовные романы
рейтинг книги
Газлайтер. Том 9
9. История Телепата
Фантастика:
фэнтези
попаданцы
рейтинг книги
Жребий некроманта 2
2. Жребий некроманта
Фантастика:
боевая фантастика
рейтинг книги
Безымянный раб
1. Дорога домой
Фантастика:
фэнтези
рейтинг книги
Я тебя не отпускал
2. Черкасовы-Ольховские
Любовные романы:
современные любовные романы
рейтинг книги
Меняя маски
1. Унесенный ветром
Фантастика:
боевая фантастика
попаданцы
рейтинг книги
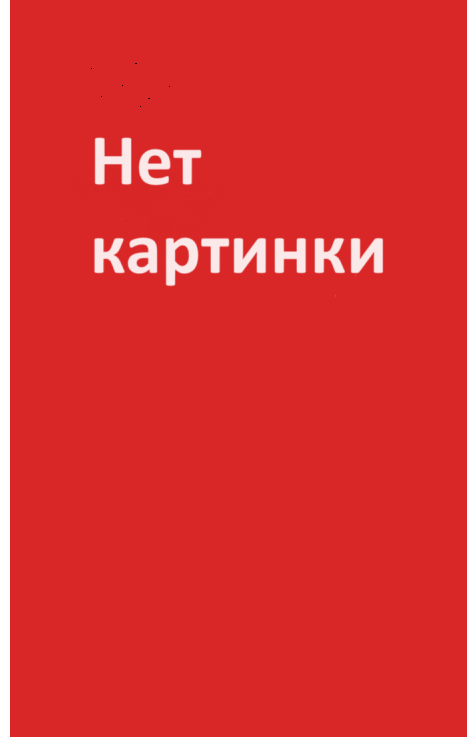